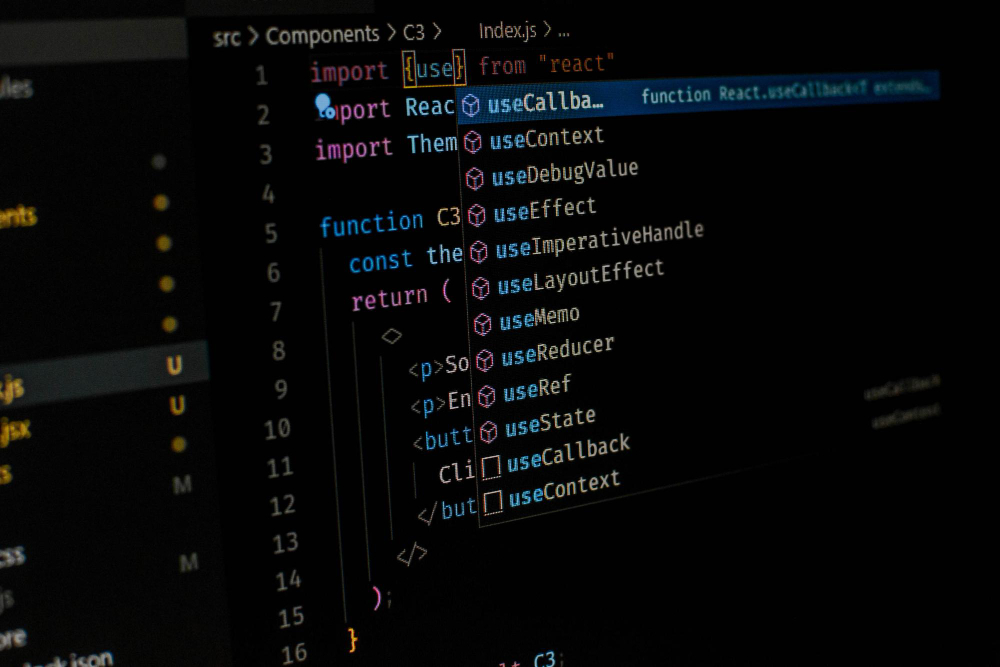
How To Check If a Key Exists In a JavaScript Object
In JavaScript, checking if a key exists in an object is a common task. Knowing how to do this efficiently can improve your code’s robustness and reliability. This article will cover several methods to perform a Check If a Key Exists In a JavaScript Object, helping you choose the best approach for your needs.
Using the in Operator
The in operator is one of the simplest ways to check if a key exists in a JavaScript object. It returns true if the key is found and false otherwise. This operator checks both the object itself and its prototype chain.
Example
const user = { name: 'John', age: 30 }; console.log('name' in user); // true console.log('address' in user); // false
The in operator is straightforward and concise, making it a popular choice for quickly checking the presence of a key.
Using hasOwnProperty Method
The hasOwnProperty method checks if the object itself has the specified key, without checking its prototype chain. This method is useful when you want to avoid keys inherited from the object's prototype.
Example
const user = { name: 'John', age: 30 }; console.log(user.hasOwnProperty('name')); // true console.log(user.hasOwnProperty('address')); // false
This method provides a reliable way to determine if a property is directly defined on an object.
Using Object.hasOwn
Introduced in ECMAScript 2022, Object.hasOwn is similar to hasOwnProperty but is more straightforward and safe for all objects, including those with null prototypes.
Example
const user = { name: 'John', age: 30 }; console.log(Object.hasOwn(user, 'name')); // true console.log(Object.hasOwn(user, 'address')); // false
Object.hasOwn simplifies the syntax and avoids potential issues with objects having null prototypes.
Using undefined Check
You can also check if a key exists by verifying if its value is undefined. However, this method can be less reliable if the object's key intentionally has an undefined value.
Example
const user = { name: 'John', age: 30, address: undefined }; console.log(user.name !== undefined); // true console.log(user.address !== undefined); // false console.log(user.phone !== undefined); // false
While straightforward, this approach can be misleading if undefined values are used in the object.
Using getOwnPropertyNames
For a more advanced approach, Object.getOwnPropertyNames can be used to get an array of the object's own properties and then check if the key is in this array.
Example
const user = { name: 'John', age: 30 }; const keys = Object.getOwnPropertyNames(user); console.log(keys.includes('name')); // true console.log(keys.includes('address')); // false
This method can be useful when you need to inspect the object's properties more thoroughly.
Using Object.keys
Another approach is using Object.keys, which returns an array of the object's own enumerable property names. You can then check if the key is in this array.
Example
const user = { name: 'John', age: 30 }; const keys = Object.keys(user); console.log(keys.includes('name')); // true console.log(keys.includes('address')); // false
Object.keys provides a quick way to get an array of keys and is often used in conjunction with array methods for more complex checks.
Using Optional Chaining (ES2020)
Optional chaining is a newer feature that allows you to check for the existence of deeply nested properties safely. If any part of the chain is null or undefined, it short-circuits and returns undefined.
Example
const user = { name: 'John', details: { address: { city: 'New York' } } }; console.log(user.details?.address?.city); // New York console.log(user.details?.address?.zip); // undefined console.log(user.contact?.email); // undefined
Optional chaining is particularly useful for avoiding errors when accessing nested properties.
Conclusion
Performing a Check If a Key Exists In a JavaScript Object is a fundamental skill for any JavaScript developer. The methods discussed—in operator, hasOwnProperty, Object.hasOwn, undefined check, Object.getOwnPropertyNames, Object.keys, and optional chaining—each have their advantages and use cases. Choose the one that best fits your specific requirements to ensure your code remains efficient and error-free.
By mastering these techniques, you can write more reliable and maintainable JavaScript code. Understanding when and how to use each method will make you a more versatile and effective programmer.